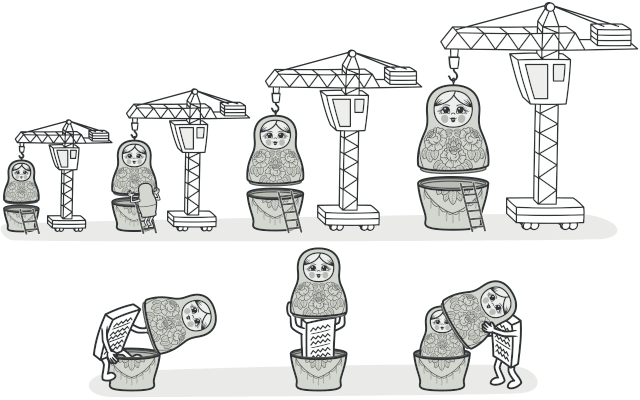
Decorator is a structural design pattern that lets you attach new behaviors to objects by placing these objects inside special wrapper objects that contain the behaviors.
Decorators have the same supertype as the objects that they decorate. You can use one or more decorators to wrap an object. The decorator adds its own behavior either before or after delegating to the object it decorates to do te work. We can decorate objects at runtime with as many decorators as we like.
Example
Problem
In this design there is a class for every combination of Beverage and Condiment.
Solution
In this design, when we add a new Beverage, the condiments don't change, or when we add a new Condiment, the beverages don't change. This follows the Decorator pattern, which respects the Open Closed Principle.
Code to create a dark roast with mocha and whip:
Beverage b = new DarkRoast( );
b = new Mocha(b);
b = new Whip(b);
We have decorated our dark roast with mocha and whip, and can use the cost( ) method, which will be delegated to the inner classes to add the individual costs.
Design principles (link):
- (OK) Single Responsibility Principle - divide a class that implements many possible variants of behavior into several smaller classes.
- (OK) Liskov Substitution Principle - adds functionality to the class it wraps without limiting it.
- (OK) Dependency Inversion Principle - the decorator extends / implements the same abstraction as the class it wraps. The client uses that abstraction.
Comments
Post a Comment